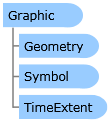
Visual Basic (Declaration) | |
---|---|
Public Class Graphic Inherits System.Windows.DependencyObject |
C# | |
---|---|
public class Graphic : System.Windows.DependencyObject |
Graphics are added dynamically to the Map, at runtime, in the client application. Graphic objects can be created in XAML or code-behind (or a combination of both).
There are several ways to add a Graphic object to a GraphicsLayer, including:
- Explicitly defining each Graphic in XAML (see the code example for the Graphic.Symbol Property)
- Explicitly defining each Graphic in code-behind (see the code example in this document)
- From the result of a Task (Query, Identify, Find, Address locator, Geometry, Geoprocessing, and Route)(see the code example for the Graphic.Attributes Property)
- From performing the Draw of a shape interactively by the user on the Map
- Loading from a file on the local hard drive (see the code example for the Graphic.MapTip Property)
- From a custom web service
Add the GraphicsLayer below (i.e. after) other Layers so the Graphics display on top.
If declaring a GraphicsLayer in XAML, provide an ID attribute in order to use it in the code-behind.
In order to display a Graphic on the Map, a Symbol must be associated with each Graphic. A Graphic’s Geometry Property must match the Symbol type. This means a MarkerSymbol for a MapPoint or MultiPoint, FillSymbol for a Polygon, and LineSymbol for a Polyline.
It is a good practice to provide a SpatialReference for the Geometry that is applied to the Graphic. Some operations like the various Geometry service functions must have a valid SpatialReference in order to work.
Special consideration for Graphics based upon Polygon geometries: In order to have the correct exterior FillSymbol.BorderBrush Symbology associated with a Polygon Graphic, it is important that the PointCollection of MapPoints that are used to define the Rings for a Polygon close back on itself. This means that the first and last MapPoint vertices must have the same coordinate values. If the first and last MapPoint vertices are not the same the Polygon graphic will close back on itself automatically but the ending segment will not have any symbology for the exterior BorderBrush displayed. See the following screen shot for a visual example:
How to use:
Click on the different RadioButtons (Point, Polyline, and Polygon) to draw Graphics generated in code-behind on the Map Control.
The XAML code in this example is used in conjunction with the code-behind (C# or VB.NET) to demonstrate the functionality.
The following screen shot corresponds to the code example in this page.
XAML | ![]() |
---|---|
<Grid x:Name="LayoutRoot"> <!-- Provide the instructions on how to use the sample code. --> <TextBlock Height="40" HorizontalAlignment="Left" Name="TextBlock1" VerticalAlignment="Top" Width="436" TextWrapping="Wrap" Margin="2,9,0,0" Text="Click on the different RadioButtons (Point, Polyline, and Polygon) to draw Graphics generated in code-behind on the Map Control." /> <!-- Add a Map control with an ArcGISTiledMapeServiceLayer. --> <esri:Map Background="White" HorizontalAlignment="Left" Margin="12,107,0,0" Name="Map1" VerticalAlignment="Top" Height="318" Width="483" > <esri:ArcGISTiledMapServiceLayer ID="PhysicalTiledLayer" Url="http://services.arcgisonline.com/ArcGIS/rest/services/World_Street_Map/MapServer" /> </esri:Map> <!-- Add three RadioButtons that use the same Click Event handlers to draw Point, Polyline, and Polygon Graphics on the Map control in the code-behind. Each RadioButton has a 'Tag' attribute set that will be used to decide which Geometry type to draw. --> <RadioButton Content="Point" Height="16" HorizontalAlignment="Left" Margin="143,82,0,0" Name="rbPoint" VerticalAlignment="Top" Tag="Point" Click="rb_Click"/> <RadioButton Content="Polyline" Height="16" HorizontalAlignment="Left" Margin="202,82,0,0" Name="rbPolyline" VerticalAlignment="Top" Tag="Polyline" Click="rb_Click"/> <RadioButton Content="Polygon" Height="16" HorizontalAlignment="Left" Margin="273,82,0,0" Name="rbPolygon" VerticalAlignment="Top" Tag="Polygon" Click="rb_Click"/> </Grid> |
C# | ![]() |
---|---|
private void rb_Click(object sender, System.Windows.RoutedEventArgs e) { // Cast the sender object to a RadioButton. System.Windows.Controls.RadioButton aRadioButton = (System.Windows.Controls.RadioButton)sender; // Get the Tag Property (a String) of the RadioButton that was set in XAML. Possible options for the Tag // Property are: "Point", "Polyline", and "Polygon". string aGraphicType = (string)aRadioButton.Tag; // Remove any existing GraphicsLayer objects that are in the Map. This allows the user to choose the // different RadioButtons over and over. if (Map1.Layers.Count > 1) { Map1.Layers.RemoveAt(1); } // Create a new instance of the GraphicsLayer object ESRI.ArcGIS.Client.GraphicsLayer myGraphicsLayer = new ESRI.ArcGIS.Client.GraphicsLayer(); // Call the function that generates a single Graphic based upon the type the user wants to create using // the RadioButton Tag and add it to the GraphicsLayer. myGraphicsLayer.Graphics.Add(GetAGraphicFromStaticSource(aGraphicType)); // Add the GraphicsLayer to the Layers collection of the Map (this will automatically cause a refresh of the Map). Map1.Layers.Add(myGraphicsLayer); } public ESRI.ArcGIS.Client.Graphic GetAGraphicFromStaticSource(string aGraphicType) { // Create a new instance of one Graphic. ESRI.ArcGIS.Client.Graphic aGraphic = new ESRI.ArcGIS.Client.Graphic(); // Generate a single Graphic based upon the user-specified type to be created. if (aGraphicType == "Point") { // Create a SpatialReference for the Graphic. ESRI.ArcGIS.Client.Geometry.SpatialReference aSpatialReference = new ESRI.ArcGIS.Client.Geometry.SpatialReference(102100); // Create a MapPoint object and set its SpatialReference and coordinate (X,Y) information. Note: Point // Graphics are known as MapPoint objects. ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint = new ESRI.ArcGIS.Client.Geometry.MapPoint(); aMapPoint.SpatialReference = aSpatialReference; aMapPoint.X = -12455356.2; aMapPoint.Y = 4978700.31; // Create a new instance of a SimpleMarkerSymbol and set its Color, Style, and Size Properties. ESRI.ArcGIS.Client.Symbols.SimpleMarkerSymbol aSimpleMarkerySymbol = new ESRI.ArcGIS.Client.Symbols.SimpleMarkerSymbol(); aSimpleMarkerySymbol.Color = new System.Windows.Media.SolidColorBrush(Colors.Red); aSimpleMarkerySymbol.Style = ESRI.ArcGIS.Client.Symbols.SimpleMarkerSymbol.SimpleMarkerStyle.Circle; aSimpleMarkerySymbol.Size = 10; // Apply the Graphic's Geometry and Symbol Properties. aGraphic.Geometry = (ESRI.ArcGIS.Client.Geometry.Geometry)aMapPoint; aGraphic.Symbol = (ESRI.ArcGIS.Client.Symbols.Symbol)aSimpleMarkerySymbol; } else if (aGraphicType == "Polyline") { // Create a SpatialReference for the Graphic. ESRI.ArcGIS.Client.Geometry.SpatialReference aSpatialReference = new ESRI.ArcGIS.Client.Geometry.SpatialReference(102100); // Create a series of MapPoint objects and set its SpatialReference and coordinate (X,Y) information. ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint1 = new ESRI.ArcGIS.Client.Geometry.MapPoint(-7356594.25, 4752385.95, aSpatialReference); ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint2 = new ESRI.ArcGIS.Client.Geometry.MapPoint(-5468910.57, 1875915.58, aSpatialReference); ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint3 = new ESRI.ArcGIS.Client.Geometry.MapPoint(-1558708.66, -326382.05, aSpatialReference); ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint4 = new ESRI.ArcGIS.Client.Geometry.MapPoint(-4614958.43, -4191639.11, aSpatialReference); // Add the MapPoint objects into a new instance of a PointCollection. ESRI.ArcGIS.Client.Geometry.PointCollection aPointCollection = new ESRI.ArcGIS.Client.Geometry.PointCollection(); aPointCollection.Add(aMapPoint1); aPointCollection.Add(aMapPoint2); aPointCollection.Add(aMapPoint3); aPointCollection.Add(aMapPoint4); // The PointCollection needs to be added to a new instance of an ObservableCollection. System.Collections.ObjectModel.ObservableCollection<ESRI.ArcGIS.Client.Geometry.PointCollection> anObservableCollection = new System.Collections.ObjectModel.ObservableCollection<ESRI.ArcGIS.Client.Geometry.PointCollection>(); anObservableCollection.Add(aPointCollection); // Create a new instance of a Polyline object and set the Paths and SpatialReference Properties. ESRI.ArcGIS.Client.Geometry.Polyline aPolyline = new ESRI.ArcGIS.Client.Geometry.Polyline(); aPolyline.Paths = anObservableCollection; aPolyline.SpatialReference = aSpatialReference; // Create a new instance of a SimpleLineSymbol and set its Color, Style, and Width Properties. ESRI.ArcGIS.Client.Symbols.SimpleLineSymbol aSimpleLineSymbol = new ESRI.ArcGIS.Client.Symbols.SimpleLineSymbol(); aSimpleLineSymbol.Color = new System.Windows.Media.SolidColorBrush(Colors.Blue); aSimpleLineSymbol.Style = ESRI.ArcGIS.Client.Symbols.SimpleLineSymbol.LineStyle.Solid; aSimpleLineSymbol.Width = 7; // Apply the Graphic's Geometry and Symbol Properties. aGraphic.Geometry = (ESRI.ArcGIS.Client.Geometry.Geometry)aPolyline; aGraphic.Symbol = (ESRI.ArcGIS.Client.Symbols.Symbol)aSimpleLineSymbol; } else if (aGraphicType == "Polygon") { // Create a SpatialReference for the Graphic. ESRI.ArcGIS.Client.Geometry.SpatialReference aSpatialReference = new ESRI.ArcGIS.Client.Geometry.SpatialReference(102100); // Create a series of MapPoint objects and set its SpatialReference and coordinate (X,Y) information. ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint1 = new ESRI.ArcGIS.Client.Geometry.MapPoint(3654893.89, 7718746.02, aSpatialReference); ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint2 = new ESRI.ArcGIS.Client.Geometry.MapPoint(1317761.71, 1741081.03, aSpatialReference); ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint3 = new ESRI.ArcGIS.Client.Geometry.MapPoint(12913532.9, -2528679.68, aSpatialReference); ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint4 = new ESRI.ArcGIS.Client.Geometry.MapPoint(14351768.09, 7359187.21, aSpatialReference); ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint5 = new ESRI.ArcGIS.Client.Geometry.MapPoint(6801033.36, 10325547.3, aSpatialReference); ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint6 = new ESRI.ArcGIS.Client.Geometry.MapPoint(3654893.89, 7718746.02, aSpatialReference); // Add the MapPoint objects into a new instance of a PointCollection. // Note: Polygons must close back on itself (i.e. the first and last MapPoint must have the same coordinates). // If the first and last MapPoint coorindates are not the same, the Polygon will it close back on itself // automatically but the ending segment will not have any symbology for the exterior BorderBrush displayed. ESRI.ArcGIS.Client.Geometry.PointCollection aPointCollection = new ESRI.ArcGIS.Client.Geometry.PointCollection(); aPointCollection.Add(aMapPoint1); aPointCollection.Add(aMapPoint2); aPointCollection.Add(aMapPoint3); aPointCollection.Add(aMapPoint4); aPointCollection.Add(aMapPoint5); aPointCollection.Add(aMapPoint6); //Comment out add the last vertex to see some interesting symbolization behavior. // The PointCollection needs to be added to a new instance of an ObservableCollection. System.Collections.ObjectModel.ObservableCollection<ESRI.ArcGIS.Client.Geometry.PointCollection> anObservableCollection = new System.Collections.ObjectModel.ObservableCollection<ESRI.ArcGIS.Client.Geometry.PointCollection>(); anObservableCollection.Add(aPointCollection); // Create a new instance of a Polygon object and set the Rings and SpatialReference Properties. ESRI.ArcGIS.Client.Geometry.Polygon aPolygon = new ESRI.ArcGIS.Client.Geometry.Polygon(); aPolygon.Rings = anObservableCollection; aPolygon.SpatialReference = aSpatialReference; // Create a new instance of a SimpleFillSymbol and set its Color, BorderBrush, and BorderThickness Properties. ESRI.ArcGIS.Client.Symbols.SimpleFillSymbol aSimpleFillSymbol = new ESRI.ArcGIS.Client.Symbols.SimpleFillSymbol(); aSimpleFillSymbol.Fill = new System.Windows.Media.SolidColorBrush(Colors.Green); aSimpleFillSymbol.BorderBrush = new System.Windows.Media.SolidColorBrush(Colors.Yellow); aSimpleFillSymbol.BorderThickness = 5; // Apply the Graphic's Geometry and Symbol Properties. aGraphic.Geometry = (ESRI.ArcGIS.Client.Geometry.Geometry)aPolygon; aGraphic.Symbol = (ESRI.ArcGIS.Client.Symbols.Symbol)aSimpleFillSymbol; } // Return the created Graphic. return aGraphic; } |
VB.NET | ![]() |
---|---|
Private Sub rb_Click(ByVal sender As System.Object, ByVal e As System.Windows.RoutedEventArgs) ' Cast the sender object to a RadioButton. Dim aRadioButton As System.Windows.Controls.RadioButton = CType(sender, System.Windows.Controls.RadioButton) ' Get the Tag Property (a String) of the RadioButton that was set in XAML. Possible options for the Tag ' Property are: "Point", "Polyline", and "Polygon". Dim aGraphicType As String = aRadioButton.Tag ' Remove any existing GraphicsLayer objects that are in the Map. This allows the user to choose the ' different RadioButtons over and over. If Map1.Layers.Count > 1 Then Map1.Layers.RemoveAt(1) End If ' Create a new instance of the GraphicsLayer object Dim myGraphicsLayer As New ESRI.ArcGIS.Client.GraphicsLayer ' Call the function that generates a single Graphic based upon the type the user wants to create using ' the RadioButton Tag and add it to the GraphicsLayer. myGraphicsLayer.Graphics.Add(GetAGraphicFromStaticSource(aGraphicType)) ' Add the GraphicsLayer to the Layers collection of the Map (this will automatically cause a refresh of the Map). Map1.Layers.Add(myGraphicsLayer) End Sub Public Function GetAGraphicFromStaticSource(ByVal aGraphicType As String) As ESRI.ArcGIS.Client.Graphic ' Create a new instance of one Graphic. Dim aGraphic As New ESRI.ArcGIS.Client.Graphic ' Generate a single Graphic based upon the user-specified type to be created. If aGraphicType = "Point" Then ' Create a SpatialReference for the Graphic. Dim aSpatialReference As New ESRI.ArcGIS.Client.Geometry.SpatialReference(102100) ' Create a MapPoint object and set its SpatialReference and coordinate (X,Y) information. Note: Point ' Graphics are known as MapPoint objects. Dim aMapPoint As New ESRI.ArcGIS.Client.Geometry.MapPoint aMapPoint.SpatialReference = aSpatialReference aMapPoint.X = -12455356.2 aMapPoint.Y = 4978700.31 ' Create a new instance of a SimpleMarkerSymbol and set its Color, Style, and Size Properties. Dim aSimpleMarkerySymbol As New ESRI.ArcGIS.Client.Symbols.SimpleMarkerSymbol aSimpleMarkerySymbol.Color = New System.Windows.Media.SolidColorBrush(Colors.Red) aSimpleMarkerySymbol.Style = ESRI.ArcGIS.Client.Symbols.SimpleMarkerSymbol.SimpleMarkerStyle.Circle aSimpleMarkerySymbol.Size = 10 ' Apply the Graphic's Geometry and Symbol Properties. aGraphic.Geometry = CType(aMapPoint, ESRI.ArcGIS.Client.Geometry.Geometry) aGraphic.Symbol = CType(aSimpleMarkerySymbol, ESRI.ArcGIS.Client.Symbols.Symbol) ElseIf aGraphicType = "Polyline" Then ' Create a SpatialReference for the Graphic. Dim aSpatialReference As New ESRI.ArcGIS.Client.Geometry.SpatialReference(102100) ' Create a series of MapPoint objects and set its SpatialReference and coordinate (X,Y) information. Dim aMapPoint1 As New ESRI.ArcGIS.Client.Geometry.MapPoint(-7356594.25, 4752385.95, aSpatialReference) Dim aMapPoint2 As New ESRI.ArcGIS.Client.Geometry.MapPoint(-5468910.57, 1875915.58, aSpatialReference) Dim aMapPoint3 As New ESRI.ArcGIS.Client.Geometry.MapPoint(-1558708.66, -326382.05, aSpatialReference) Dim aMapPoint4 As New ESRI.ArcGIS.Client.Geometry.MapPoint(-4614958.43, -4191639.11, aSpatialReference) ' Add the MapPoint objects into a new instance of a PointCollection. Dim aPointCollection As New ESRI.ArcGIS.Client.Geometry.PointCollection aPointCollection.Add(aMapPoint1) aPointCollection.Add(aMapPoint2) aPointCollection.Add(aMapPoint3) aPointCollection.Add(aMapPoint4) ' The PointCollection needs to be added to a new instance of an ObservableCollection. Dim anObservableCollection As New System.Collections.ObjectModel.ObservableCollection(Of ESRI.ArcGIS.Client.Geometry.PointCollection) anObservableCollection.Add(aPointCollection) ' Create a new instance of a Polyline object and set the Paths and SpatialReference Properties. Dim aPolyline As New ESRI.ArcGIS.Client.Geometry.Polyline aPolyline.Paths = anObservableCollection aPolyline.SpatialReference = aSpatialReference ' Create a new instance of a SimpleLineSymbol and set its Color, Style, and Width Properties. Dim aSimpleLineSymbol As New ESRI.ArcGIS.Client.Symbols.SimpleLineSymbol aSimpleLineSymbol.Color = New System.Windows.Media.SolidColorBrush(Colors.Blue) aSimpleLineSymbol.Style = ESRI.ArcGIS.Client.Symbols.SimpleLineSymbol.LineStyle.Solid aSimpleLineSymbol.Width = 7 ' Apply the Graphic's Geometry and Symbol Properties. aGraphic.Geometry = CType(aPolyline, ESRI.ArcGIS.Client.Geometry.Geometry) aGraphic.Symbol = CType(aSimpleLineSymbol, ESRI.ArcGIS.Client.Symbols.Symbol) ElseIf aGraphicType = "Polygon" Then ' Create a SpatialReference for the Graphic. Dim aSpatialReference As New ESRI.ArcGIS.Client.Geometry.SpatialReference(102100) ' Create a series of MapPoint objects and set its SpatialReference and coordinate (X,Y) information. Dim aMapPoint1 As New ESRI.ArcGIS.Client.Geometry.MapPoint(3654893.89, 7718746.02, aSpatialReference) Dim aMapPoint2 As New ESRI.ArcGIS.Client.Geometry.MapPoint(1317761.71, 1741081.03, aSpatialReference) Dim aMapPoint3 As New ESRI.ArcGIS.Client.Geometry.MapPoint(12913532.9, -2528679.68, aSpatialReference) Dim aMapPoint4 As New ESRI.ArcGIS.Client.Geometry.MapPoint(14351768.09, 7359187.21, aSpatialReference) Dim aMapPoint5 As New ESRI.ArcGIS.Client.Geometry.MapPoint(6801033.36, 10325547.3, aSpatialReference) Dim aMapPoint6 As New ESRI.ArcGIS.Client.Geometry.MapPoint(3654893.89, 7718746.02, aSpatialReference) ' Add the MapPoint objects into a new instance of a PointCollection. ' Note: Polygons must close back on itself (i.e. the first and last MapPoint must have the same coordinates). ' If the first and last MapPoint coorindates are not the same, the Polygon will it close back on itself ' automatically but the ending segment will not have any symbology for the exterior BorderBrush displayed. Dim aPointCollection As New ESRI.ArcGIS.Client.Geometry.PointCollection aPointCollection.Add(aMapPoint1) aPointCollection.Add(aMapPoint2) aPointCollection.Add(aMapPoint3) aPointCollection.Add(aMapPoint4) aPointCollection.Add(aMapPoint5) aPointCollection.Add(aMapPoint6) 'Comment out add the last vertex to see some interesting symbolization behavior. ' The PointCollection needs to be added to a new instance of an ObservableCollection. Dim anObservableCollection As New System.Collections.ObjectModel.ObservableCollection(Of ESRI.ArcGIS.Client.Geometry.PointCollection) anObservableCollection.Add(aPointCollection) ' Create a new instance of a Polygon object and set the Rings and SpatialReference Properties. Dim aPolygon As New ESRI.ArcGIS.Client.Geometry.Polygon aPolygon.Rings = anObservableCollection aPolygon.SpatialReference = aSpatialReference ' Create a new instance of a SimpleFillSymbol and set its Color, BorderBrush, and BorderThickness Properties. Dim aSimpleFillSymbol As New ESRI.ArcGIS.Client.Symbols.SimpleFillSymbol aSimpleFillSymbol.Fill = New System.Windows.Media.SolidColorBrush(Colors.Green) aSimpleFillSymbol.BorderBrush = New System.Windows.Media.SolidColorBrush(Colors.Yellow) aSimpleFillSymbol.BorderThickness = 5 ' Apply the Graphic's Geometry and Symbol Properties. aGraphic.Geometry = CType(aPolygon, ESRI.ArcGIS.Client.Geometry.Geometry) aGraphic.Symbol = CType(aSimpleFillSymbol, ESRI.ArcGIS.Client.Symbols.Symbol) End If ' Return the created Graphic. Return aGraphic End Function |
System.Object
System.Windows.DependencyObject
ESRI.ArcGIS.Client.Graphic
Target Platforms: Windows XP Professional, Windows Server 2003 family, Windows Vista, Windows Server 2008 family, Windows 7