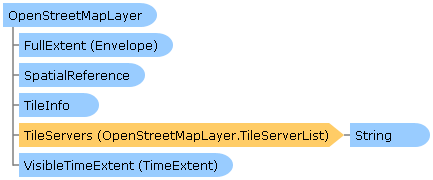
Visual Basic (Declaration) | |
---|---|
Public Class OpenStreetMapLayer Inherits WebTiledLayer Implements ESRI.ArcGIS.Client.IAttribution |
C# | |
---|---|
public class OpenStreetMapLayer : WebTiledLayer, ESRI.ArcGIS.Client.IAttribution |
OpenStreetMap is an organization that provides free world-wide tiled based map services to the public. OpenStreetMapLayers are specialized layers that consume web services using the OpenStreetMap specification. A tiled map service means that map images are pre-created on a server to improve drawing performance in a client application. Because the images are pre-created cached tiles, re-projection on-the-fly to a different SpatialReference is not possible.
By generating pre-created cached tiles and storing them in an optimized file structure on a server using the OpenStreetMap specification, client applications can see improved draw times of geographic phenomena. This performance increase comes as a trade-off of not having the flexibility to dynamically change the drawing of geographic phenomena on-the-fly. This means that an OpenStreetMapLayer does not have Properties like dynamic map service layers such as the ArcGISDynamicMapServiceLayer or a FeatureLayer have for things such as: LayerDefinition, Where, TimeExtent, and/or VisibleLayers which restrict which features are returned.
A call to the OpenStreetMapLayer is Asynchronous. As a result, this means that obtaining information (i.e. get/Read) for the various Properties of an OpenStreetMapLayer should occur in the Initialized Event or any time after the Initialized Event occurs. This ensures that information retrieved about the OpenStreetMapLayer has been obtained after a complete round trip from the server. Do not be tempted to try and access OpenStreetMapLayer Property information from generic application Events like: MainWindows.Loaded or the Constructor, etc. as the OpenStreetMapLayer has not been Initialized and erroneous information will be returned. Likewise, OpenStreetMapLayer Methods should not be invoked until after the OpenStreetMapLayer Initialized Event has fired or from within the Initialized Event to avoid erroneous results.
It is highly recommended to use the OpenStreetMapLayer InitializationFailed Event to test for valid data being returned from the Server. Some common reasons for an OpenStreetMapLayer failing to initialize include the server being down or an incorrect Url was specified in the OpenStreetMapLayer.TileServers Property. If proper error handling is not done in the OpenStreetMapLayer InitializationFailed Event, an 'Unhandled Exception' error message will be thrown by Visual Studio causing undesirable application termination.
It is only required to create a new instance of an OpenStreetMapLayer and then add it to the Map.Layers Property to display a default OpenStreetMapLayer. The reason for this is that the internals of the OpenStreetMapLayer constructor automatically uses an internal Url to a web service provided by the OpenStreetMap organization. So the following is an XAML example of all that is needed to create a default OpenStreetMapLayer:
<esri:Map x:Name="MyMap"> <esri:OpenStreetMapLayer /> </esri:Map>
The OpenStreetMap organization hosts several types of maps that can be used as OpenStreetMapLayer's. To change which type of map is used, specify the OpenStreetMapLayer.Style Property to any one of several OpenStreetMapLayer.MapStyle Enumerations. The default OpenStreetMap.MapStyle Property is OpenStreetMapLayer.MapStyle.Mapnik meaning that if an OpenStreetMapLayer.Style is not specified in constructing an OpenStreetMapLayer, the OpenStreetMapLayer.MapStyle.Mapnik style will be used by default. The following is an XAML example of specifying a specific OpenStreetMapLayer.Style when defining a new OpenStreetMapLayer:
<esri:Map x:Name="MyMap"> <esri:OpenStreetMapLayer Style=”CycleMap”/> </esri:Map>
If it is not desired to use the map services provided directly by the OpenStreetMap organization or if you discover that additional map services are provided for which Esri has not provided an explicit OpenStreetMapLayer.Style, developers can explicitly provide their own Url's for an OpenStreetMapLayer using the OpenStreetMapLayer.TileServers Property. When using the OpenStreetMapLayer.TileServers Property, the OpenStreetMapLayer.Style Property is ignored. The OpenStreetMapLayer.TileServerList Class is a List Collection of Strings that are the Url's to various OpenStreetMap map based servers. The following is an XAML example of specifying specific Urls using the OpenStreetMapLayer.TileServers Property when defining a new OpenStreetMapLayer:
<esri:Map x:Name="MyMap"> <esri:OpenStreetMapLayer ID="osmLayer"> <esri:OpenStreetMapLayer.TileServers> <sys:String>http://otile1.mqcdn.com/tiles/1.0.0/osm</sys:String> <sys:String>http://otile2.mqcdn.com/tiles/1.0.0/osm</sys:String> <sys:String>http://otile3.mqcdn.com/tiles/1.0.0/osm</sys:String> </esri:OpenStreetMapLayer.TileServers> </esri:OpenStreetMapLayer> </esri:Map>
It is important to understand that only one Url is needed in the OpenStreetMapLayer.TileServerList. If multiple Urls are included in the OpenStreetMapLayer.TileServerList, all of the map servers should be serving up the same base data. The reason for having the ability to specify multiple Urls in the OpenStreetMapLayer.TileServerList is to improve performance by load balancing the requests the client application uses across multiple servers. If different OpenStreetMap based map services are specified in the OpenStreetMapLayer.TileServerList, the tiles that are placed together in the Esri Map control will yield unexpected results. For example, assume that three different OpenStreetMap map based services are used for the the OpenStreetMapLayer.TileServers Property in the following XAML example code:
<esri:Map x:Name="MyMap"> <esri:OpenStreetMapLayer ID="osmLayer"> <esri:OpenStreetMapLayer.TileServers> <sys:String>http://otile1.mqcdn.com/tiles/1.0.0/osm</sys:String> <sys:String>http://a.tile.openstreetmap.org</sys:String> <sys:String>http://a.tile.opencyclemap.org/cycle</sys:String> </esri:OpenStreetMapLayer.TileServers> </esri:OpenStreetMapLayer> </esri:Map>
The following is a screen shot of the previous XAML code fragment showing the application's undesirable results appearing if different map based services were used in the the OpenStreetMap.TileServers Property:
Whenever an OpenStreetMapLayer is used in a production application based on services from the OpenStreetMap organization, it is required by their license agreement to provide the appropriate credit for using their data. The credit information for maps provided by the OpenStreetMap organization is stored in the WebTiledLayer.CopyrightText Property. To display the credit information in your application is most easily accomplished by adding an Esri Attribution control to your client application and binding the Attribution.Layers Property to the OpenStreetMapLayer. The following is an XAML example of how to accomplish this:
<esri:Map x:Name="MyMap"> <esri:OpenStreetMapLayer Style="Mapnik" /> </esri:Map> <esri:Attribution Layers="{Binding ElementName=MyMap, Path=Layers}" />
OpenStreetMap is released under the Create Commons "Attribution-Share Alike 2.0 Generic" license.
How to use:
Click the Button to add an OpenStreetMapLayer to the Map (it will be added via code-behind). The credit information about the dataset will displayed in the Attribution Control.
The XAML code in this example is used in conjunction with the code-behind (C# or VB.NET) to demonstrate the functionality.
The following screen shot corresponds to the code example in this page.
XAML | ![]() |
---|---|
<Grid x:Name="LayoutRoot" > <!-- Add a Map Control to the application. --> <esri:Map x:Name="Map1" WrapAround="True" HorizontalAlignment="Left" VerticalAlignment="Top" Margin="0,156,0,0" Height="350" Width="550" /> <!-- Add an Attribution Control. --> <esri:Attribution x:Name="Attribution1" Width="550" Height="50" HorizontalAlignment="Left" Margin="0,512,0,38" /> <!-- Add a Button that will allow the user to add an OpenStreetMapLayer via code-behind. --> <Button Name="Button1" Height="23" HorizontalAlignment="Left" Margin="0,128,0,0" VerticalAlignment="Top" Width="550" Content="Add an OpenStreetMapLayer (via code-behind)." Click="Button1_Click" /> <!-- Provide the instructions on how to use the sample code. --> <TextBlock Height="122" HorizontalAlignment="Left" Name="TextBlock1" VerticalAlignment="Top" Width="572" TextWrapping="Wrap" Text="Click the Button to add an OpenStreetMapLayer to the Map (it will be added via code-behind). The credit information about the dataset will displayed in the Attribution Control." /> </Grid> |
C# | ![]() |
---|---|
private void Button1_Click(object sender, System.Windows.RoutedEventArgs e) { // Create a new instance of an OpenStreetMapLayer. ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer myOpenStreetMapLayer = new ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer(); // Set the OpenStreetMapLayer.Style to that of MapStyle.CycleMap myOpenStreetMapLayer.Style = ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer.MapStyle.CycleMap; // Wire up an Initialized Event handler for the OpenStreetMapLayer. myOpenStreetMapLayer.Initialized += myOpenStreetMapLayer_Initialized; // Add the OpenStreetMapLayer to the Map's Layer Collection. This will cause the OpenStreetMapLayer.Initialized Event to fire. Map1.Layers.Add(myOpenStreetMapLayer); } private void myOpenStreetMapLayer_Initialized(object sender, EventArgs e) { // Get the OpenStreetMapLayer. ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer myOpenStreetMapLayer = (ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer)sender; // Display the OpenStreetMapLayer credit information via the ESRI Attribution Control. Attribution1.Layers = Map1.Layers; } |
VB.NET | ![]() |
---|---|
Private Sub Button1_Click(sender As System.Object, e As System.Windows.RoutedEventArgs) ' Create a new instance of an OpenStreetMapLayer. Dim myOpenStreetMapLayer As ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer = New ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer ' Set the OpenStreetMapLayer.Style to that of MapStyle.CycleMap myOpenStreetMapLayer.Style = ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer.MapStyle.CycleMap ' Wire up an Initialized Event handler for the OpenStreetMapLayer. AddHandler myOpenStreetMapLayer.Initialized, AddressOf myOpenStreetMapLayer_Initialized ' Add the OpenStreetMapLayer to the Map's Layer Collection. This will cause the OpenStreetMapLayer.Initialized Event to fire. Map1.Layers.Add(myOpenStreetMapLayer) End Sub Private Sub myOpenStreetMapLayer_Initialized(sender As Object, e As EventArgs) ' Get the OpenStreetMapLayer. Dim myOpenStreetMapLayer As ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer = sender ' Display the OpenStreetMapLayer credit information via the ESRI Attribution Control. Attribution1.Layers = Map1.Layers End Sub |
System.Object
System.Windows.Threading.DispatcherObject
System.Windows.DependencyObject
ESRI.ArcGIS.Client.Layer
ESRI.ArcGIS.Client.TiledLayer
ESRI.ArcGIS.Client.TiledMapServiceLayer
ESRI.ArcGIS.Client.Toolkit.DataSources.WebTiledLayer
ESRI.ArcGIS.Client.Toolkit.DataSources.OpenStreetMapLayer
Target Platforms: Windows XP Professional, Windows Server 2003 family, Windows Vista, Windows Server 2008 family, Windows 7, Windows 8