Constructs a point in the polar coordinate system defined by baseLine and its 'from' point. The angle is in radians.
[Visual Basic .NET] Public Sub ConstructDeflection ( _ ByVal baseLine As ILine, _ ByVal distance As Double, _ ByVal inAngle As Double _ )
[C#] public void ConstructDeflection ( ILine baseLine, double distance, double inAngle );
[C++]
HRESULT ConstructDeflection(
ILine* baseLine,
double distance,
double inAngle
);
[C++]Parameters
baseLinebaseLine is a parameter of type ILine
distance distance is a parameter of type double inAngle inAngle is a parameter of type double
Product Availability
Description
The ConstructDeflection method, given a line (baseline), creates a new point at a distance (distance) and at an angle (inAngle).
Remarks
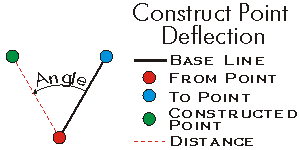
//Constructs a point from the baseline from (0,0) to (1,1)
// with the deflection angle of 45 degrees (PI/4 radians).
public void ConstructDeflection()
{
IPoint fromPoint = new PointClass();
fromPoint.PutCoords(0, 0);
IPoint toPoint = new PointClass();
toPoint.PutCoords(1, 1);
ILine line = new LineClass();
line.PutCoords(fromPoint, toPoint);
double distance = 1.4142135623731;
double angle = Math.PI / 4;
IConstructPoint constructionPoint = new PointClass();
constructionPoint.ConstructDeflection(line, distance, angle);
IPoint point = constructionPoint as IPoint;
System.Windows.Forms.MessageBox.Show("x,y = " + point.X + ", " + point.Y);
}
'+++ Constructs a point from the baseline from (0,0) to (1,1)
'+++ with the deflection angle of 45 degrees (PI/4 radians).
Public Sub t_ConstructDeflection()
On Error GoTo Errorhandler
Dim pPointFrom As IPoint
Dim pPointTo As IPoint
Dim pLine As ILine
Dim pPoint As IPoint
Dim pCPoint As IConstructPoint
Dim dDist As Double
Dim dAngle As Double
Dim pi As Double
pPointFrom = New Point
pPointTo = New Point
pCPoint = New Point
pPoint = New Point
pLine = New Line
pPointFrom.PutCoords(0, 0)
pPointTo.PutCoords(1, 1)
pLine.PutCoords(pPointFrom, pPointTo)
dDist = 1.4142135623731
pi = 4 * Math.Atan(1)
dAngle = pi / 4
pCPoint.ConstructDeflection(pLine, dDist, dAngle)
pPoint = pCPoint
MsgBox("x,y = " & pPoint.X & "," & pPoint.Y)
Exit Sub
Errorhandler:
MsgBox(Err.Number & "..." & Err.Description)
Exit Sub
End Sub